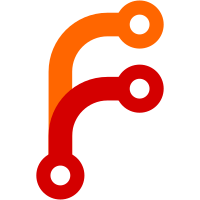
git-svn-id: svn://sunsvr01.isp.uni-luebeck.de/swproj13/trunk@609 72836036-5685-4462-b002-a69064685172
95 lines
2.3 KiB
Java
95 lines
2.3 KiB
Java
package jrummikub.control.network;
|
|
|
|
import static org.junit.Assert.*;
|
|
|
|
import java.awt.Color;
|
|
|
|
import jrummikub.control.turn.ITurnControl.TurnInfo;
|
|
import jrummikub.control.turn.TurnMode;
|
|
import jrummikub.model.GameSettings;
|
|
import jrummikub.model.GameState;
|
|
import jrummikub.model.PlayerSettings;
|
|
import jrummikub.model.PlayerSettings.Type;
|
|
import jrummikub.model.Position;
|
|
import jrummikub.model.RoundState;
|
|
import jrummikub.model.Stone;
|
|
import jrummikub.model.StoneColor;
|
|
import jrummikub.model.StoneSet;
|
|
import jrummikub.model.Table;
|
|
import jrummikub.util.IListener;
|
|
import jrummikub.util.Pair;
|
|
import jrummikub.view.MockView;
|
|
|
|
import org.junit.Before;
|
|
import org.junit.Test;
|
|
|
|
/** */
|
|
public class NetworkTurnControlTest {
|
|
private MockConnectionControl connectionControl;
|
|
private MockView view;
|
|
|
|
private GameSettings gameSettings;
|
|
private RoundState roundState;
|
|
|
|
private NetworkTurnControl turnControl;
|
|
|
|
private boolean fired;
|
|
|
|
/** */
|
|
@Before
|
|
public void setup() {
|
|
gameSettings = new GameSettings();
|
|
|
|
gameSettings.getPlayerList().add(new PlayerSettings("Ida", Color.RED));
|
|
gameSettings.getPlayerList().get(0).setType(Type.NETWORK);
|
|
gameSettings.getPlayerList().add(
|
|
new PlayerSettings("Matthias", Color.YELLOW));
|
|
gameSettings.getPlayerList().get(1).setType(Type.NETWORK);
|
|
|
|
roundState = new RoundState(gameSettings, new GameState());
|
|
|
|
view = new MockView();
|
|
connectionControl = new MockConnectionControl();
|
|
turnControl = new NetworkTurnControl(connectionControl);
|
|
}
|
|
|
|
/** */
|
|
@Test
|
|
public void testTableUpdate() {
|
|
turnControl.setup(new TurnInfo(roundState, TurnMode.NORMAL_TURN, true),
|
|
gameSettings, view);
|
|
turnControl.startTurn();
|
|
|
|
Table table = new Table(gameSettings);
|
|
StoneSet set = new StoneSet(new Stone(StoneColor.RED));
|
|
|
|
table.drop(set, new Position(0, 0));
|
|
connectionControl.tableUpdateEvent.emit(table);
|
|
|
|
int n = 0;
|
|
for (Pair<StoneSet, Position> entry : view.tablePanel.stoneSets) {
|
|
assertSame(entry.getFirst(), set);
|
|
n++;
|
|
}
|
|
assertEquals(1, n);
|
|
}
|
|
|
|
/** */
|
|
@Test
|
|
public void testRedeal() {
|
|
turnControl.getRedealEvent().add(new IListener() {
|
|
@Override
|
|
public void handle() {
|
|
fired = true;
|
|
}
|
|
});
|
|
|
|
turnControl.setup(new TurnInfo(roundState, TurnMode.MAY_REDEAL, true),
|
|
gameSettings, view);
|
|
turnControl.startTurn();
|
|
|
|
connectionControl.redealEvent.emit();
|
|
|
|
assertTrue(fired);
|
|
}
|
|
}
|