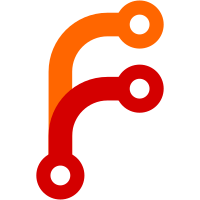
git-svn-id: svn://sunsvr01.isp.uni-luebeck.de/swproj13/trunk@579 72836036-5685-4462-b002-a69064685172
169 lines
4.7 KiB
Java
169 lines
4.7 KiB
Java
package jrummikub.view.impl;
|
|
|
|
import java.awt.Color;
|
|
import java.awt.Dimension;
|
|
import java.awt.GridBagConstraints;
|
|
import java.awt.GridBagLayout;
|
|
import java.awt.event.ActionEvent;
|
|
import java.awt.event.ActionListener;
|
|
|
|
import javax.swing.Box;
|
|
import javax.swing.JButton;
|
|
import javax.swing.JLabel;
|
|
import javax.swing.JPanel;
|
|
import javax.swing.JPasswordField;
|
|
import javax.swing.JTextField;
|
|
import javax.swing.border.CompoundBorder;
|
|
import javax.swing.border.EmptyBorder;
|
|
import javax.swing.border.LineBorder;
|
|
|
|
import jrummikub.util.Event;
|
|
import jrummikub.util.Event1;
|
|
import jrummikub.util.IEvent;
|
|
import jrummikub.util.IEvent1;
|
|
import jrummikub.util.LoginData;
|
|
import jrummikub.view.ILoginPanel;
|
|
|
|
@SuppressWarnings("serial")
|
|
class LoginPanel extends JPanel implements ILoginPanel {
|
|
private Event1<LoginData> loginEvent = new Event1<LoginData>();
|
|
private Event cancelEvent = new Event();
|
|
private Event1<String> useDedicatedServer = new Event1<String>();
|
|
private JTextField userNameField;
|
|
private JTextField serverNameField;
|
|
private JTextField passwordField;
|
|
private JTextField channelNameField;
|
|
private JButton startDedicatedServerButton;
|
|
private static final String DEFAULT_PASSWORD = "jrummikub";
|
|
|
|
LoginPanel() {
|
|
setLayout(new GridBagLayout());
|
|
GridBagConstraints c = new GridBagConstraints();
|
|
c.fill = GridBagConstraints.BOTH;
|
|
c.gridwidth = GridBagConstraints.REMAINDER;
|
|
c.weightx = 1;
|
|
c.weighty = 1;
|
|
|
|
ActionListener loginAction = createInputFields();
|
|
|
|
c.weighty = 0;
|
|
add(startDedicatedServerButton, c);
|
|
startDedicatedServerButton.addActionListener(new ActionListener() {
|
|
@Override
|
|
public void actionPerformed(ActionEvent arg0) {
|
|
useDedicatedServer.emit(passwordField.getText().isEmpty() ? DEFAULT_PASSWORD
|
|
: passwordField.getText());
|
|
}
|
|
});
|
|
|
|
c.weighty = 1;
|
|
add(Box.createVerticalGlue(), c);
|
|
|
|
c.gridwidth = 1;
|
|
c.weighty = 0;
|
|
JButton loginButton = new JButton("Login");
|
|
loginButton.addActionListener(loginAction);
|
|
add(loginButton, c);
|
|
|
|
c.weightx = 0;
|
|
add(Box.createHorizontalStrut(10), c);
|
|
|
|
c.gridwidth = GridBagConstraints.REMAINDER;
|
|
c.weightx = 1;
|
|
JButton cancelButton = new JButton("Abbrechen");
|
|
cancelButton.addActionListener(new ActionListener() {
|
|
@Override
|
|
public void actionPerformed(ActionEvent arg0) {
|
|
cancelEvent.emit();
|
|
}
|
|
});
|
|
add(cancelButton, c);
|
|
|
|
setBorder(new CompoundBorder(new LineBorder(Color.BLACK),
|
|
new EmptyBorder(10, 10, 10, 10)));
|
|
}
|
|
|
|
private ActionListener createInputFields() {
|
|
ActionListener loginAction = new ActionListener() {
|
|
@Override
|
|
public void actionPerformed(ActionEvent arg0) {
|
|
login();
|
|
}
|
|
};
|
|
|
|
userNameField = addInputRow("Benutzername:", new JTextField());
|
|
userNameField.addActionListener(loginAction);
|
|
serverNameField = addInputRow("Server:", new JTextField());
|
|
serverNameField.addActionListener(loginAction);
|
|
passwordField = addInputRow("Passwort:", new JPasswordField());
|
|
passwordField.addActionListener(loginAction);
|
|
channelNameField = addInputRow("Channel:", new JTextField());
|
|
channelNameField.addActionListener(loginAction);
|
|
startDedicatedServerButton = new JButton("Dedizierten Server starten");
|
|
// this fixes some strange layouting bug
|
|
startDedicatedServerButton.setPreferredSize(new Dimension(500, 10));
|
|
return loginAction;
|
|
}
|
|
|
|
void resetLoginPanel() {
|
|
// TODO leer machen
|
|
userNameField.setText("test1");
|
|
serverNameField.setText("universe-factory.net");
|
|
passwordField.setText("");
|
|
channelNameField.setText("jrummikub@muc.universe-factory.net");
|
|
}
|
|
|
|
@Override
|
|
public IEvent1<LoginData> getLoginEvent() {
|
|
return loginEvent;
|
|
}
|
|
|
|
@Override
|
|
public IEvent getCancelEvent() {
|
|
return cancelEvent;
|
|
}
|
|
|
|
@Override
|
|
public IEvent1<String> getUseDedicatedServerEvent() {
|
|
return useDedicatedServer;
|
|
}
|
|
|
|
private JTextField addInputRow(String label, JTextField textField) {
|
|
GridBagConstraints c = new GridBagConstraints();
|
|
c.fill = GridBagConstraints.HORIZONTAL;
|
|
c.gridwidth = 1;
|
|
c.weightx = 1;
|
|
c.weighty = 0;
|
|
|
|
add(new JLabel(label), c);
|
|
|
|
c.weightx = 1;
|
|
c.fill = GridBagConstraints.HORIZONTAL;
|
|
c.gridwidth = GridBagConstraints.REMAINDER;
|
|
add(textField, c);
|
|
return textField;
|
|
}
|
|
|
|
private void login() {
|
|
loginEvent.emit(new LoginData(userNameField.getText(), serverNameField
|
|
.getText(), passwordField.getText().isEmpty() ? DEFAULT_PASSWORD
|
|
: passwordField.getText(), channelNameField.getText()));
|
|
}
|
|
|
|
@Override
|
|
public void setServer(String server) {
|
|
serverNameField.setText(server);
|
|
}
|
|
|
|
@Override
|
|
public void setChannel(String channel) {
|
|
channelNameField.setText(channel);
|
|
}
|
|
|
|
@Override
|
|
public void setDedicatedServerRunning(boolean running) {
|
|
startDedicatedServerButton
|
|
.setText(running ? "Dedizierten Server nutzen"
|
|
: "Dedizierten Server starten");
|
|
}
|
|
}
|