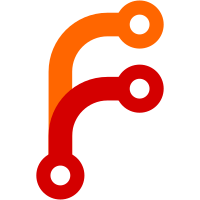
git-svn-id: svn://sunsvr01.isp.uni-luebeck.de/swproj13/trunk@531 72836036-5685-4462-b002-a69064685172
83 lines
1.5 KiB
Java
83 lines
1.5 KiB
Java
package jrummikub.model;
|
|
|
|
import java.util.ArrayList;
|
|
import java.util.HashMap;
|
|
import java.util.Iterator;
|
|
import java.util.List;
|
|
import java.util.Map;
|
|
|
|
import jrummikub.util.Pair;
|
|
|
|
/**
|
|
* Mock class for {@link Table}
|
|
*/
|
|
@SuppressWarnings("serial")
|
|
public class MockTable implements ITable {
|
|
/** */
|
|
public Map<Stone, StoneSet> findStoneSet = new HashMap<Stone, StoneSet>();
|
|
/** */
|
|
public boolean valid = false;
|
|
/** */
|
|
public ITable clonedTable;
|
|
/** */
|
|
public List<Pair<StoneSet, Position>> sets = new ArrayList<Pair<StoneSet, Position>>();
|
|
|
|
@Override
|
|
public void pickUpStone(Stone stone) {
|
|
// TODO: Auto-generated method stub
|
|
}
|
|
|
|
@Override
|
|
public boolean isValid() {
|
|
return valid;
|
|
}
|
|
|
|
@Override
|
|
public void drop(StoneSet object, Position position) {
|
|
sets.add(new Pair<StoneSet, Position>(object, position));
|
|
}
|
|
|
|
@Override
|
|
public Position getPosition(StoneSet object) {
|
|
// TODO Auto-generated method stub
|
|
return null;
|
|
}
|
|
|
|
@Override
|
|
public boolean pickUp(StoneSet object) {
|
|
return false;
|
|
}
|
|
|
|
@Override
|
|
public Iterator<Pair<StoneSet, Position>> iterator() {
|
|
return sets.iterator();
|
|
}
|
|
|
|
@Override
|
|
public StoneSet findStoneSet(Stone stone) {
|
|
return findStoneSet.get(stone);
|
|
}
|
|
|
|
public ITable clone() {
|
|
if (clonedTable == null)
|
|
return this;
|
|
return clonedTable;
|
|
}
|
|
|
|
@Override
|
|
public int getSize() {
|
|
return sets.size();
|
|
}
|
|
|
|
@Override
|
|
public boolean contains(StoneSet object) {
|
|
for (Pair<StoneSet, Position> set : sets) {
|
|
if (set.getFirst() == object) {
|
|
return true;
|
|
}
|
|
}
|
|
|
|
return false;
|
|
}
|
|
|
|
}
|