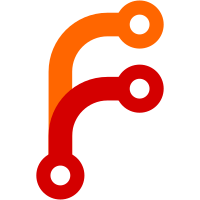
git-svn-id: svn://sunsvr01.isp.uni-luebeck.de/swproj13/trunk@547 72836036-5685-4462-b002-a69064685172
225 lines
5.5 KiB
Java
225 lines
5.5 KiB
Java
package jrummikub.view.impl;
|
|
|
|
import java.awt.Color;
|
|
import java.awt.Dimension;
|
|
import java.awt.Graphics;
|
|
import java.awt.Graphics2D;
|
|
import java.awt.Insets;
|
|
import java.awt.RenderingHints;
|
|
import java.awt.image.BufferedImage;
|
|
import java.util.Collection;
|
|
import java.util.Collections;
|
|
|
|
import javax.swing.ImageIcon;
|
|
import javax.swing.border.MatteBorder;
|
|
|
|
import jrummikub.model.Hand;
|
|
import jrummikub.model.Position;
|
|
import jrummikub.model.Stone;
|
|
import jrummikub.util.Pair;
|
|
import jrummikub.view.IHandPanel;
|
|
import jrummikub.view.impl.StonePainter.StoneState;
|
|
|
|
/**
|
|
* Implementation of the board
|
|
*/
|
|
@SuppressWarnings("serial")
|
|
class HandPanel extends AbstractStonePanel implements IHandPanel {
|
|
private final static int HEIGHT = 2;
|
|
|
|
private final static BufferedImage BACKGROUND;
|
|
private final static BufferedImage DARK_BACKGROUND;
|
|
static {
|
|
ImageIcon image = new ImageIcon(
|
|
HandPanel.class.getResource("/jrummikub/resource/wood.png"));
|
|
ImageIcon darkImage = new ImageIcon(
|
|
HandPanel.class
|
|
.getResource("/jrummikub/resource/dark_wood.png"));
|
|
BACKGROUND = new BufferedImage(image.getIconWidth(),
|
|
image.getIconHeight(), BufferedImage.TYPE_INT_RGB);
|
|
DARK_BACKGROUND = new BufferedImage(darkImage.getIconWidth(),
|
|
darkImage.getIconHeight(), BufferedImage.TYPE_INT_RGB);
|
|
|
|
image.paintIcon(null, BACKGROUND.createGraphics(), 0, 0);
|
|
darkImage.paintIcon(null, DARK_BACKGROUND.createGraphics(), 0, 0);
|
|
}
|
|
|
|
private BufferedImage scaledBackground = BACKGROUND;
|
|
private BufferedImage scaledDarkBackground = BACKGROUND;
|
|
|
|
private PlayerPanel playerPanel;
|
|
|
|
private int currentRow = 0;
|
|
private int maxRow = 0;
|
|
|
|
private boolean repaintAll = true;
|
|
private Collection<Stone> selectedStones = Collections.emptyList();
|
|
|
|
/**
|
|
* Creates a new Board instance
|
|
*/
|
|
HandPanel(PlayerPanel playerPanel) {
|
|
this.playerPanel = playerPanel;
|
|
|
|
setBorder(new MatteBorder(0, 1, 0, 1, Color.DARK_GRAY));
|
|
}
|
|
|
|
private BufferedImage getScaledBackground(int size, BufferedImage background) {
|
|
BufferedImage scaled = new BufferedImage(size, size,
|
|
BufferedImage.TYPE_INT_RGB);
|
|
Graphics2D g = scaled.createGraphics();
|
|
g.setRenderingHint(RenderingHints.KEY_INTERPOLATION,
|
|
RenderingHints.VALUE_INTERPOLATION_BICUBIC);
|
|
|
|
g.drawImage(background, 0, 0, size, size, null);
|
|
|
|
return scaled;
|
|
}
|
|
|
|
@Override
|
|
protected void paintComponent(Graphics g1) {
|
|
Insets insets = getInsets();
|
|
int x = insets.left, y = insets.top, width = getWidth() - insets.left
|
|
- insets.right, height = getHeight() - insets.top
|
|
- insets.bottom;
|
|
Graphics2D g = (Graphics2D) g1.create(x, y, width, height);
|
|
|
|
int size = height / HEIGHT;
|
|
|
|
BufferedImage background = isEnabled() ? scaledBackground
|
|
: scaledDarkBackground;
|
|
|
|
if (repaintAll) {
|
|
if (background.getHeight() != size) {
|
|
if (!isEnabled()) {
|
|
scaledDarkBackground = getScaledBackground(size,
|
|
DARK_BACKGROUND);
|
|
} else {
|
|
scaledBackground = getScaledBackground(size, BACKGROUND);
|
|
}
|
|
background = isEnabled() ? scaledBackground
|
|
: scaledDarkBackground;
|
|
}
|
|
|
|
for (int i = 0; i < HEIGHT; ++i) {
|
|
for (int xpos = -size * i / 3; xpos < width; xpos += size) {
|
|
g.drawImage(background, xpos, size * i, null);
|
|
}
|
|
}
|
|
}
|
|
|
|
Pair<Integer, Integer> trans = getTranslation();
|
|
g.translate(trans.getFirst(), trans.getSecond());
|
|
|
|
for (Pair<Stone, Position> entry : getStones()) {
|
|
getStonePainter()
|
|
.paintStone(
|
|
g,
|
|
entry.getFirst(),
|
|
entry.getSecond(),
|
|
selectedStones.contains(entry.getFirst()) ? StoneState.SELECTED
|
|
: StoneState.NORMAL,
|
|
entry.getFirst() == getHoveredStone());
|
|
}
|
|
}
|
|
|
|
@Override
|
|
public void setStones(Iterable<Pair<Stone, Position>> stones) {
|
|
super.setStones(stones);
|
|
|
|
maxRow = 0;
|
|
for (Pair<Stone, Position> entry : stones) {
|
|
if (entry.getSecond().getY() > maxRow) {
|
|
maxRow = (int) entry.getSecond().getY();
|
|
}
|
|
}
|
|
|
|
repaintAll = true;
|
|
repaint();
|
|
|
|
playerPanel.updateButtons();
|
|
}
|
|
|
|
/**
|
|
* Updates panel after visible row changed
|
|
*/
|
|
void rowUp() {
|
|
currentRow--;
|
|
repaintAll = true;
|
|
repaint();
|
|
|
|
playerPanel.updateButtons();
|
|
}
|
|
|
|
/**
|
|
* Updates panel after visible row changed
|
|
*/
|
|
void rowDown() {
|
|
currentRow++;
|
|
repaintAll = true;
|
|
repaint();
|
|
|
|
playerPanel.updateButtons();
|
|
}
|
|
|
|
@Override
|
|
public void resetCurrentRow() {
|
|
currentRow = Math.max(0, maxRow - 1);
|
|
|
|
playerPanel.updateButtons();
|
|
}
|
|
|
|
/**
|
|
* Is there a higher row than the current row
|
|
*
|
|
* @return higher row present
|
|
*/
|
|
boolean canRowUp() {
|
|
return (currentRow > 0);
|
|
}
|
|
|
|
/**
|
|
* Is there a lower row than the current row
|
|
*
|
|
* @return higher row present
|
|
*/
|
|
boolean canRowDown() {
|
|
return (currentRow < maxRow);
|
|
}
|
|
|
|
@Override
|
|
public Pair<Integer, Integer> getTranslation() {
|
|
return new Pair<Integer, Integer>(0, -getStonePainter()
|
|
.getStoneHeight() * currentRow);
|
|
}
|
|
|
|
/**
|
|
* Sets the stones that are to be painted selected
|
|
*
|
|
* @param stones
|
|
* the selected stones
|
|
*/
|
|
void setSelectedStones(Collection<Stone> stones) {
|
|
selectedStones = stones;
|
|
repaint();
|
|
}
|
|
|
|
/**
|
|
* Rescales the hand panel according to window size
|
|
*
|
|
* @param height
|
|
* of hand panel
|
|
* @return width of hand panel
|
|
*/
|
|
int rescale(int height) {
|
|
Insets insets = getInsets();
|
|
int size = (height - insets.top - insets.bottom) / HEIGHT;
|
|
getStonePainter().setScale(size * StonePainter.HEIGHT_SCALE);
|
|
int width = Hand.WIDTH * getStonePainter().getStoneWidth()
|
|
+ insets.left + insets.right;
|
|
setSize(new Dimension(width, getHeight()));
|
|
repaintAll = true;
|
|
return width;
|
|
}
|
|
|
|
}
|