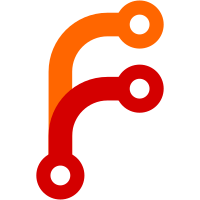
git-svn-id: svn://sunsvr01.isp.uni-luebeck.de/swproj13/trunk@85 72836036-5685-4462-b002-a69064685172
72 lines
1.8 KiB
Java
72 lines
1.8 KiB
Java
package jrummikub.model;
|
|
|
|
import java.util.Arrays;
|
|
import java.util.Collections;
|
|
|
|
import org.junit.*;
|
|
|
|
import static jrummikub.model.StoneColor.BLACK;
|
|
import static jrummikub.model.StoneColor.RED;
|
|
import static org.junit.Assert.*;
|
|
|
|
import jrummikub.model.Table;
|
|
|
|
public class TableTest {
|
|
Table testTable;
|
|
|
|
@Before
|
|
public void setup() {
|
|
testTable = new Table();
|
|
}
|
|
|
|
@Test
|
|
public void testIsValid() {
|
|
testTable.drop(new StoneSet(Arrays.asList(new Stone(RED), new Stone(BLACK),
|
|
new Stone(1, BLACK))), new Position(0,0));
|
|
testTable.drop(new StoneSet(Arrays.asList(new Stone(1, RED), new Stone(2, RED),
|
|
new Stone(3, RED))), new Position(0,0));
|
|
assertTrue(testTable.isValid());
|
|
|
|
testTable.drop(new StoneSet(Arrays.asList(new Stone(5, RED))), new Position(0,0));
|
|
assertFalse(testTable.isValid());
|
|
}
|
|
|
|
@Test
|
|
public void testEmptyIsValid() {
|
|
assertTrue(testTable.isValid());
|
|
}
|
|
|
|
@Test
|
|
@SuppressWarnings("unused")
|
|
public void testPickUpStoneGroup() {
|
|
Stone targetStone = new Stone(BLACK);
|
|
testTable.drop(new StoneSet(Arrays.asList(new Stone(RED), targetStone,
|
|
new Stone(1, BLACK))), new Position(0,0));
|
|
assertTrue(testTable.isValid());
|
|
testTable.pickUpStone(targetStone);
|
|
assertFalse(testTable.isValid());
|
|
|
|
int counter = 0;
|
|
for (Object i : testTable) {
|
|
counter++;
|
|
}
|
|
assertEquals(counter, 1);
|
|
}
|
|
|
|
@Test
|
|
@SuppressWarnings("unused")
|
|
public void testPickUpStoneRun() {
|
|
Stone targetStone = new Stone(BLACK);
|
|
testTable.drop(new StoneSet(Arrays.asList(new Stone(1, RED), targetStone,
|
|
new Stone(3, BLACK))), new Position(0,0));
|
|
assertTrue(testTable.isValid());
|
|
testTable.pickUpStone(targetStone);
|
|
assertFalse(testTable.isValid());
|
|
|
|
int counter = 0;
|
|
for (Object i : testTable) {
|
|
counter++;
|
|
}
|
|
assertEquals(counter, 2);
|
|
}
|
|
}
|