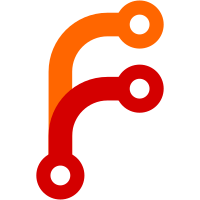
turn git-svn-id: svn://sunsvr01.isp.uni-luebeck.de/swproj13/trunk@273 72836036-5685-4462-b002-a69064685172
111 lines
No EOL
2.1 KiB
Java
111 lines
No EOL
2.1 KiB
Java
package jrummikub.model;
|
|
|
|
/**
|
|
* Interface for {@link RoundState} model
|
|
*/
|
|
public interface IRoundState {
|
|
|
|
/**
|
|
* Get the current {@link GameSettings}
|
|
*
|
|
* @return The game settings
|
|
*/
|
|
public GameSettings getGameSettings();
|
|
|
|
/**
|
|
* Get the current {@link Table}
|
|
*
|
|
* @return The current Table
|
|
*/
|
|
public ITable getTable();
|
|
|
|
/**
|
|
* Sets the current {@link Table}
|
|
*
|
|
* @param table
|
|
* The new Table
|
|
*/
|
|
public void setTable(ITable table);
|
|
|
|
/**
|
|
* Returns the number of players
|
|
*
|
|
* @return number of players
|
|
*/
|
|
public int getPlayerCount();
|
|
|
|
/** Changes the activePlayer to the next {@link Player} in the list */
|
|
public void nextPlayer();
|
|
|
|
/**
|
|
* Returns the currently active player
|
|
*
|
|
* @return currently active player
|
|
*/
|
|
public IPlayer getActivePlayer();
|
|
|
|
/**
|
|
* Returns the heap of stones to draw from
|
|
*
|
|
* @return heap of stones
|
|
*/
|
|
public StoneHeap getGameHeap();
|
|
|
|
/**
|
|
* Returns the player that would be the active player after i turns
|
|
*
|
|
* @param i
|
|
* number of turns
|
|
* @return player active after i turns
|
|
*/
|
|
public IPlayer getNthNextPlayer(int i);
|
|
|
|
/**
|
|
* Returns the nth player
|
|
*
|
|
* @param i
|
|
* player number
|
|
* @return nth player
|
|
*/
|
|
public IPlayer getNthPlayer(int i);
|
|
|
|
/**
|
|
* Sets the player that will make the last turn before the round ends when
|
|
* the heap is empty
|
|
*
|
|
* @return the last player
|
|
*/
|
|
public abstract IPlayer getLastPlayer();
|
|
|
|
/**
|
|
* Gets the player that will make the last turn before the round ends when
|
|
* the heap is empty
|
|
*
|
|
* @param lastPlayer
|
|
* the last player
|
|
*/
|
|
public abstract void setLastPlayer(IPlayer lastPlayer);
|
|
|
|
/**
|
|
* Makes the player with number i the active player
|
|
*
|
|
* @param i
|
|
* number of the player to make active
|
|
*/
|
|
public void setActivePlayerNumber(int i);
|
|
|
|
/**
|
|
* Gets the number of the current turn. Numbers smaller than one indicate
|
|
* hand inspection turns
|
|
*
|
|
* @return current turn number
|
|
*/
|
|
public abstract int getTurnNumber();
|
|
|
|
/**
|
|
* Increments the turn number
|
|
*
|
|
*/
|
|
public void nextTurn();
|
|
|
|
} |