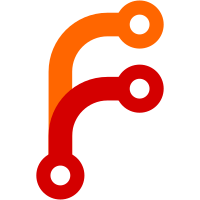
git-svn-id: svn://sunsvr01.isp.uni-luebeck.de/swproj13/trunk@484 72836036-5685-4462-b002-a69064685172
140 lines
3 KiB
Java
140 lines
3 KiB
Java
package jrummikub.control;
|
|
|
|
import java.io.File;
|
|
import java.io.FileInputStream;
|
|
import java.io.FileOutputStream;
|
|
import java.io.ObjectInputStream;
|
|
import java.io.ObjectOutputStream;
|
|
|
|
import jrummikub.model.GameSettings;
|
|
import jrummikub.model.GameState;
|
|
import jrummikub.model.IRoundState;
|
|
import jrummikub.util.Event;
|
|
import jrummikub.util.Event3;
|
|
import jrummikub.util.IEvent3;
|
|
import jrummikub.util.IListener1;
|
|
import jrummikub.view.IView;
|
|
|
|
/**
|
|
* The save control is responsible for loading and saving game and round states
|
|
*/
|
|
public class SaveControl {
|
|
private GameSettings gameSettings;
|
|
private GameState gameState;
|
|
private IRoundState roundState;
|
|
private Event3<GameSettings, GameState, IRoundState> loadEvent = new Event3<GameSettings, GameState, IRoundState>();
|
|
private Event loadErrorEvent = new Event();
|
|
|
|
/**
|
|
* Creates a new SaveControl
|
|
*
|
|
* @param view
|
|
* the view to use
|
|
*/
|
|
public SaveControl(IView view) {
|
|
view.getSaveEvent().add(new IListener1<File>() {
|
|
@Override
|
|
public void handle(File file) {
|
|
save(file);
|
|
}
|
|
});
|
|
|
|
view.getLoadEvent().add(new IListener1<File>() {
|
|
@Override
|
|
public void handle(File file) {
|
|
load(file);
|
|
}
|
|
});
|
|
}
|
|
|
|
/**
|
|
* Getter for loadEvent
|
|
*
|
|
* @return loadEvent
|
|
*/
|
|
public IEvent3<GameSettings, GameState, IRoundState> getLoadEvent() {
|
|
return loadEvent;
|
|
}
|
|
|
|
/**
|
|
* Sets the current game settings
|
|
*
|
|
* @param gameSettings
|
|
* the game settings
|
|
*/
|
|
public void setGameSettings(GameSettings gameSettings) {
|
|
this.gameSettings = gameSettings;
|
|
}
|
|
|
|
/**
|
|
* Sets the current game state
|
|
*
|
|
* @param gameState
|
|
* the game state
|
|
*/
|
|
public void setGameState(GameState gameState) {
|
|
this.gameState = gameState;
|
|
}
|
|
|
|
/**
|
|
* Sets the current round state
|
|
*
|
|
* @param roundState
|
|
* the round state
|
|
*/
|
|
public void setRoundState(IRoundState roundState) {
|
|
this.roundState = roundState;
|
|
}
|
|
|
|
private void load(File file) {
|
|
try {
|
|
ObjectInputStream stream = new ObjectInputStream(
|
|
new FileInputStream(file));
|
|
|
|
gameSettings = (GameSettings) stream.readObject();
|
|
gameState = (GameState) stream.readObject();
|
|
roundState = (IRoundState) stream.readObject();
|
|
|
|
stream.close();
|
|
|
|
if (gameState == null || gameSettings == null) {
|
|
loadErrorEvent.emit();
|
|
return;
|
|
}
|
|
|
|
loadEvent.emit(gameSettings, gameState, roundState);
|
|
|
|
} catch (Exception e) {
|
|
loadErrorEvent.emit();
|
|
}
|
|
}
|
|
|
|
/**
|
|
* The load error event is emitted when the file selected for loading is not
|
|
* a rum file
|
|
*
|
|
* @return the event
|
|
*/
|
|
public Event getLoadErrorEvent() {
|
|
return loadErrorEvent;
|
|
}
|
|
|
|
private void save(File file) {
|
|
if (gameState == null || gameSettings == null) {
|
|
return;
|
|
}
|
|
try {
|
|
ObjectOutputStream stream = new ObjectOutputStream(
|
|
new FileOutputStream(file));
|
|
|
|
stream.writeObject(gameSettings);
|
|
stream.writeObject(gameState);
|
|
stream.writeObject(roundState);
|
|
stream.flush();
|
|
|
|
stream.close();
|
|
} catch (Exception e) {
|
|
e.printStackTrace();
|
|
}
|
|
}
|
|
}
|