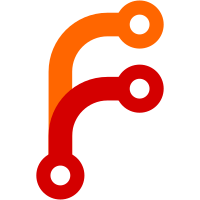
git-svn-id: svn://sunsvr01.isp.uni-luebeck.de/swproj13/trunk@426 72836036-5685-4462-b002-a69064685172
165 lines
3.9 KiB
Java
165 lines
3.9 KiB
Java
package jrummikub.control.network;
|
|
|
|
import java.util.ArrayList;
|
|
import java.util.HashMap;
|
|
import java.util.List;
|
|
import java.util.Map;
|
|
import java.util.UUID;
|
|
|
|
import jrummikub.model.GameSettings;
|
|
import jrummikub.util.Connection;
|
|
import jrummikub.util.Event;
|
|
import jrummikub.util.GameData;
|
|
import jrummikub.util.IEvent;
|
|
import jrummikub.util.IListener;
|
|
import jrummikub.util.IListener1;
|
|
import jrummikub.util.LoginData;
|
|
import jrummikub.view.IView;
|
|
|
|
/**
|
|
* Class dealing with network connection, offering and choice of network games
|
|
*/
|
|
public class NetworkControl {
|
|
private ConnectionControl connectionControl;
|
|
private IView view;
|
|
private List<Connection> connections = new ArrayList<Connection>();
|
|
private Event stopNetworkEvent = new Event();
|
|
|
|
private NetworkSettingsControl settingsControl;
|
|
|
|
private Map<UUID, GameData> gameMap = new HashMap<UUID, GameData>();
|
|
|
|
/**
|
|
* Creates a new network control
|
|
*
|
|
* @param loginData
|
|
* user's login data
|
|
* @param view
|
|
* for events and handlers
|
|
*/
|
|
public NetworkControl(final LoginData loginData, final IView view) {
|
|
this.view = view;
|
|
connectionControl = new ConnectionControl(loginData);
|
|
|
|
addConnectionControlListeners(loginData, view);
|
|
|
|
connections.add(view.getGameListPanel().getJoinEvent()
|
|
.add(new IListener1<GameData>() {
|
|
@Override
|
|
public void handle(GameData value) {
|
|
// TODO Auto-generated method stub
|
|
|
|
}
|
|
}));
|
|
|
|
connections.add(view.getGameListPanel().getOpenNewGameEvent()
|
|
.add(new IListener() {
|
|
@Override
|
|
public void handle() {
|
|
if (settingsControl == null) {
|
|
view.showGameListPanel(false);
|
|
|
|
settingsControl = new NetworkSettingsControl(
|
|
connectionControl.getNickname(), view, new GameSettings());
|
|
settingsControl.startSettings();
|
|
}
|
|
}
|
|
}));
|
|
|
|
connections.add(view.getGameListPanel().getCancelEvent()
|
|
.add(new IListener() {
|
|
@Override
|
|
public void handle() {
|
|
abort();
|
|
stopNetworkEvent.emit();
|
|
}
|
|
}));
|
|
}
|
|
|
|
/**
|
|
* Adds the listeners for connection control events
|
|
*
|
|
* @param loginData
|
|
* player's login data
|
|
* @param view
|
|
* view for events
|
|
*/
|
|
public void addConnectionControlListeners(final LoginData loginData,
|
|
final IView view) {
|
|
connections.add(connectionControl.getConnectedEvent().add(
|
|
new IListener() {
|
|
@Override
|
|
public void handle() {
|
|
view.getGameListPanel().setChannelName(
|
|
loginData.getChannelName());
|
|
view.showGameListPanel(true);
|
|
}
|
|
}));
|
|
|
|
connections.add(connectionControl.getConnectionFailedEvent().add(
|
|
new IListener() {
|
|
@Override
|
|
public void handle() {
|
|
// TODO Auto-generated method stub
|
|
|
|
}
|
|
}));
|
|
|
|
connections.add(connectionControl.getGameOfferEvent().add(
|
|
new IListener1<GameData>() {
|
|
@Override
|
|
public void handle(GameData value) {
|
|
GameData game = gameMap.get(value.getGameID());
|
|
|
|
if (game == null) {
|
|
game = value;
|
|
gameMap.put(value.getGameID(), value);
|
|
} else {
|
|
game.setGameSettings(value.getGameSettings());
|
|
}
|
|
|
|
view.getGameListPanel().addGame(game);
|
|
}
|
|
}));
|
|
connections.add(connectionControl.getGameWithdrawalEvent().add(
|
|
new IListener1<UUID>() {
|
|
@Override
|
|
public void handle(UUID value) {
|
|
GameData game = gameMap.get(value);
|
|
|
|
if (game != null) {
|
|
view.getGameListPanel().removeGame(game);
|
|
gameMap.remove(value);
|
|
}
|
|
}
|
|
}));
|
|
}
|
|
|
|
/**
|
|
* Starts a new network connection with the sepcified data
|
|
*/
|
|
public void startNetwork() {
|
|
connectionControl.connect();
|
|
}
|
|
|
|
/**
|
|
* Ends the network connection if canceled
|
|
*/
|
|
public void abort() {
|
|
for (Connection c : connections) {
|
|
c.remove();
|
|
}
|
|
connectionControl.disconnect();
|
|
view.showGameListPanel(false);
|
|
}
|
|
|
|
/**
|
|
* Getter for stopNetworkEvent
|
|
*
|
|
* @return stopNetworkEvent
|
|
*/
|
|
public IEvent getStopNetworkEvent() {
|
|
return stopNetworkEvent;
|
|
}
|
|
|
|
}
|