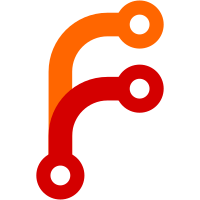
git-svn-id: svn://sunsvr01.isp.uni-luebeck.de/swproj13/trunk@286 72836036-5685-4462-b002-a69064685172
97 lines
1.9 KiB
Java
97 lines
1.9 KiB
Java
package jrummikub.view;
|
|
|
|
import java.util.Collection;
|
|
|
|
import jrummikub.model.Stone;
|
|
import jrummikub.util.IEvent;
|
|
|
|
/**
|
|
* The top-level view interface
|
|
*/
|
|
public interface IView {
|
|
/**
|
|
* Returns the settings panel
|
|
*
|
|
* @return the settings panel
|
|
*/
|
|
public ISettingsPanel getSettingsPanel();
|
|
|
|
/**
|
|
* Returns the table
|
|
*
|
|
* @return the table
|
|
*/
|
|
public ITablePanel getTablePanel();
|
|
|
|
/**
|
|
* @return the board where the players hand stones are displayed
|
|
*/
|
|
public IHandPanel getHandPanel();
|
|
|
|
/**
|
|
* Returns the player panel
|
|
*
|
|
* @return the playerPanel
|
|
*/
|
|
public IPlayerPanel getPlayerPanel();
|
|
|
|
/**
|
|
* Sets the current player's name
|
|
*
|
|
* @param playerName
|
|
* the player name
|
|
*/
|
|
public void setCurrentPlayerName(String playerName);
|
|
|
|
/**
|
|
* Sets the stones that are to be painted selected
|
|
*
|
|
* @param stones
|
|
* the stones to be painted selected
|
|
*/
|
|
public void setSelectedStones(Collection<Stone> stones);
|
|
|
|
/**
|
|
* Enables or disables the player's StartTurnPanel
|
|
*
|
|
* @param enable
|
|
* enable/disable
|
|
*/
|
|
public void enableStartTurnPanel(boolean enable);
|
|
|
|
/**
|
|
* The start turn event is emitted when the player wants to start his turn
|
|
*
|
|
* @return the event
|
|
*/
|
|
public IEvent getStartTurnEvent();
|
|
|
|
/**
|
|
* Enables or disables the panel shown when a player has won
|
|
*
|
|
* @param enable
|
|
* enable/disable
|
|
*/
|
|
void enableWinPanel(boolean enable);
|
|
|
|
/**
|
|
* The quit event is emitted when the player wants to quit the game
|
|
*
|
|
* @return the event
|
|
*/
|
|
public IEvent getFinalScoreEvent();
|
|
|
|
/**
|
|
* The new round event is emitted when the player wants to start a new round
|
|
*
|
|
* @return the event
|
|
*/
|
|
IEvent getNewRoundEvent();
|
|
|
|
/**
|
|
* Shows or hides the game settings panel
|
|
*
|
|
* @param show specifies if the panel shall be shown or hidden
|
|
*/
|
|
void showSettingsPanel(boolean show);
|
|
}
|